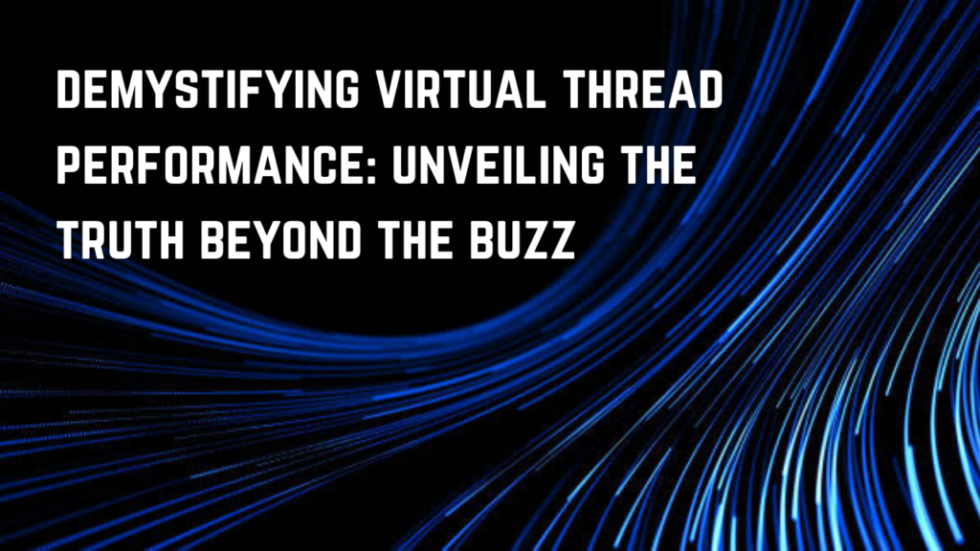
Virtual threads have become a buzzword in the tech industry, often hailed as the next big step in concurrency programming. But what exactly are virtual threads, and do they live up to the hype? This article aims to break down the intricacies of virtual thread performance, exploring their benefits, limitations, and real-world applications. By the end, you’ll have a comprehensive understanding of what virtual threads bring to the table and whether they’re worth the attention they’ve garnered.
Understanding Virtual Threads
Virtual threads are lightweight threads introduced to simplify concurrency and parallelism in programming, particularly in Java’s Project Loom. Unlike traditional threads managed by the operating system (OS), virtual threads are managed by the Java Virtual Machine (JVM). This approach aims to provide high performance with minimal resource consumption.
Key Features of Virtual Threads:
- Lightweight Nature: Virtual threads consume fewer system resources compared to OS threads.
- Simplified Concurrency: They abstract away much of the complexity involved in multithreaded programming.
- Scalability: Thousands or even millions of virtual threads can run concurrently on a single JVM.
- Blocking Compatibility: Virtual threads allow blocking operations to be used without significant performance penalties.
How Virtual Threads Work
Virtual threads operate differently from traditional threads. They’re decoupled from OS threads, leveraging a shared pool of underlying OS threads. This means multiple virtual threads can share the same OS thread, reducing the overhead of thread context switching and improving scalability.
The Lifecycle of a Virtual Thread:
- Creation: Virtual threads are created similarly to traditional threads but without the associated OS-level cost.
- Execution: When a virtual thread performs a blocking operation, it’s automatically unmounted from the OS thread, freeing the latter for other tasks.
- Completion: Once the blocking operation is complete, the virtual thread is remounted on an available OS thread.
Advantages of Virtual Threads
- Enhanced Performance:
- Traditional threads are expensive to create and maintain due to their reliance on OS resources. Virtual threads, by contrast, significantly reduce this cost.
- Improved Scalability:
- Virtual threads allow developers to scale applications without worrying about running out of thread resources. This is particularly beneficial for high-concurrency applications like web servers.
- Easier Debugging:
- Virtual threads simplify debugging by reducing the complexity of thread management. Developers can write synchronous code that performs like asynchronous code.
- Compatibility with Existing Code:
- One of the most significant advantages of virtual threads is their compatibility with existing Java code, allowing for seamless integration.
Limitations of Virtual Threads
While virtual threads offer numerous benefits, they’re not without their limitations:
- Learning Curve:
- Developers accustomed to traditional thread models may need time to adapt to virtual threads.
- Resource Contention:
- While virtual threads are lightweight, they still compete for underlying hardware resources, which could lead to bottlenecks in certain scenarios.
- JVM Dependency:
- Virtual threads are tied to the JVM, making them less versatile for non-Java environments.
- Not a Silver Bullet:
- Virtual threads excel in high-concurrency scenarios but may not significantly impact applications with low thread counts.
Real-World Applications of Virtual Threads
Web Servers:
Virtual threads are particularly beneficial for web servers handling thousands of concurrent requests. For instance, frameworks like Spring Boot and Quarkus have started integrating virtual thread support to improve scalability and reduce resource usage.
Microservices:
In microservices architectures, where services handle numerous lightweight tasks, virtual threads can optimize resource utilization and simplify code.
Big Data Processing:
Big data applications often require parallel processing of large datasets. Virtual threads enable efficient utilization of hardware resources, improving processing speeds.
Performance Benchmarks
Virtual Threads vs. Traditional Threads:
The following table provides a detailed comparison of virtual threads and traditional threads based on key performance metrics:
Feature/Spec | Virtual Threads | Traditional Threads |
---|---|---|
Thread Creation Time | Minimal (few microseconds) | High (milliseconds) |
Memory Consumption | Low (few KB per thread) | High (1 MB per thread) |
Concurrency | Millions of threads possible | Limited by OS resources |
Context Switching | Low overhead | High overhead |
Blocking Operations | Efficient (unmount/remount model) | Resource-intensive |
Ease of Debugging | High | Moderate |
Compatibility with JVM | Native | Native |
Case Study: Implementing Virtual Threads in a Web Application
Consider a scenario where a web server needs to handle 100,000 concurrent HTTP requests. Using traditional threads, the server would struggle due to high memory consumption and thread management overhead. With virtual threads, each request can be handled by its own lightweight thread, ensuring efficient resource utilization and better performance.
Code Example:
public class VirtualThreadExample {
public static void main(String[] args) {
try (var executor = Executors.newVirtualThreadPerTaskExecutor()) {
IntStream.range(0, 100_000).forEach(i ->
executor.submit(() -> System.out.println("Task " + i))
);
}
}
}
This code demonstrates the simplicity of creating 100,000 virtual threads to handle tasks concurrently.
Future of Virtual Threads
The introduction of virtual threads marks a significant milestone in programming. As adoption increases, we can expect:
- Better Tooling:
- Improved debugging and monitoring tools tailored for virtual threads.
- Enhanced Framework Support:
- More frameworks integrating native support for virtual threads.
- Wider Adoption Beyond Java:
- Other languages may adopt similar concepts to improve concurrency models.
- Optimization for Multicore Processors:
- Virtual threads will likely become even more efficient as processors with higher core counts become standard.
Conclusion
Virtual threads offer a groundbreaking approach to concurrency, addressing many limitations of traditional threads. By leveraging their lightweight nature and scalability, developers can build high-performance applications that handle millions of concurrent tasks seamlessly. While not a universal solution, virtual threads represent a significant step forward, particularly for high-concurrency applications.
Whether you’re developing a web server, a microservice, or a data processing pipeline, understanding virtual thread performance can help you make informed decisions about their integration into your projects. As the ecosystem around virtual threads continues to evolve, they’re poised to become an integral part of modern software development.
FAQs
Q1: Are virtual threads suitable for all applications? A: No, virtual threads are best suited for high-concurrency scenarios. Applications with low concurrency demands may not benefit significantly.
Q2: How do virtual threads handle blocking I/O operations? A: Virtual threads unmount themselves from OS threads during blocking operations, freeing the OS thread for other tasks.
Q3: Can I use virtual threads in non-Java environments? A: Currently, virtual threads are a Java-specific feature tied to the JVM.
Q4: Are there any downsides to using virtual threads? A: Potential downsides include a learning curve for developers and dependency on the JVM.
Q5: How can I monitor virtual thread performance? A: Tools like JDK Flight Recorder and VisualVM can help monitor and debug virtual thread performance.