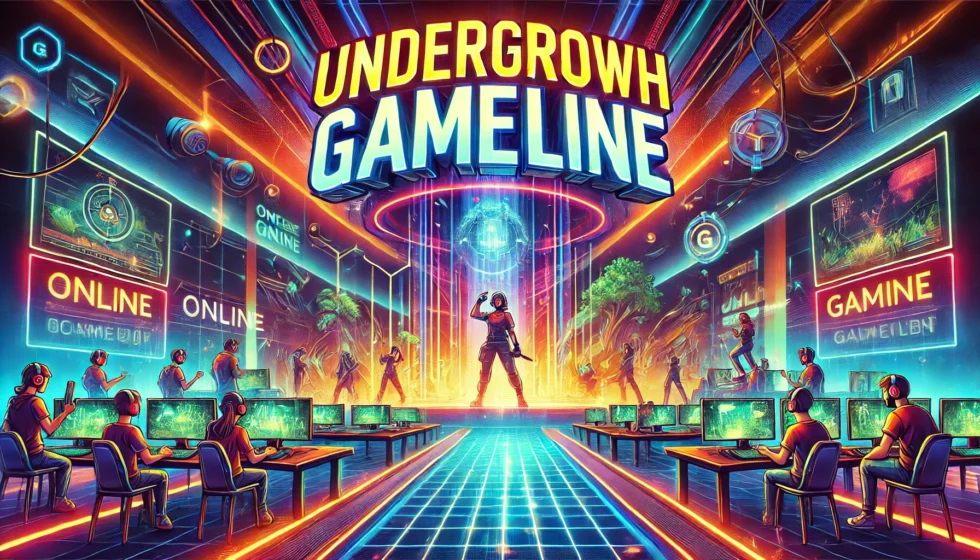
In the vast and evolving world of software development, coding is often romanticized as an art form where creativity meets logic. Yet, beneath the surface lies a set of unspoken rules that distinguish great developers from the rest. These rules transcend programming languages and frameworks, offering invaluable insights for both novice coders and seasoned developers. Understanding and embracing these principles can enhance productivity, foster collaboration, and build robust, scalable applications.
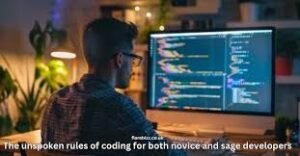
the unspoken rules of coding for both novice and sage developers
1. Code is Communication
While the primary purpose of code is to instruct computers, its secondary, yet equally important, role is to communicate with other developers (including your future self). Writing clean, readable code ensures that your work is maintainable and understandable.
- For Novices: Focus on naming variables, functions, and classes descriptively. Avoid cryptic abbreviations or single-letter names unless their meaning is universally understood.
- For Veterans: Strive for self-documenting code. Use comments sparingly but effectively to explain complex logic. Remember, clarity trumps cleverness.
Example of clean code:
# Calculate the total price of items in a shopping cart
def calculate_total(cart_items):
total_price = 0
for item in cart_items:
total_price += item['price'] * item['quantity']
return total_price
2. Respect the DRY Principle (Don’t Repeat Yourself)
Redundancy is the enemy of efficient coding. The DRY principle encourages developers to minimize code duplication, making applications easier to maintain and less prone to bugs.
- For Novices: Identify repetitive patterns and learn how to encapsulate them into functions or classes.
- For Veterans: Regularly refactor code to eliminate redundancies. Consider using design patterns to streamline complex structures.
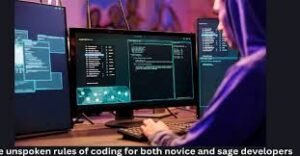
3. Embrace Version Control
Version control systems like Git are indispensable for modern developers. They enable collaboration, track changes, and provide a safety net when things go awry.
- For Novices: Learn the basics of Git, such as cloning repositories, committing changes, and creating branches.
- For Veterans: Adopt advanced practices like rebasing, squashing commits, and using hooks. Always write meaningful commit messages.
Read More=The Ultimate Guide to UndergrowthGameLine Hosted Events
4. Error Handling is Non-Negotiable
Errors are inevitable in programming, but how you handle them defines the quality of your software.
- For Novices: Learn to use try-catch blocks or equivalent error-handling mechanisms. Provide informative error messages.
- For Veterans: Implement logging systems and create custom error classes. Ensure that your error handling doesn’t reveal sensitive information.
Example of error handling:
try:
file = open('data.txt', 'r')
data = file.read()
except FileNotFoundError:
print("File not found. Please check the path.")
finally:
file.close()
5. Test, Then Test Some More
Testing ensures that your code behaves as expected under various conditions.
- For Novices: Start with manual testing and learn the basics of unit testing frameworks like JUnit (Java), PyTest (Python), or Jest (JavaScript).
- For Veterans: Incorporate integration and end-to-end testing. Write automated test suites and aim for high test coverage.
6. Never Underestimate Documentation
Comprehensive documentation is the backbone of any successful project.
- For Novices: Document your code as you write it. Use tools like Markdown or JSDoc to create basic documentation.
- For Veterans: Maintain detailed API documentation and user guides. Use tools like Swagger or Sphinx for structured documentation.
7. Collaboration is Key
Coding is rarely a solo endeavor. Teamwork and communication are crucial for successful development.
- For Novices: Participate in code reviews and pair programming sessions to learn from others.
- For Veterans: Mentor junior developers and foster an environment of open communication and constructive feedback.
8. Keep Security in Mind
Security is not an afterthought—it’s a fundamental part of coding.
- For Novices: Avoid hardcoding sensitive information. Learn about basic security practices, like sanitizing inputs.
- For Veterans: Conduct regular security audits and keep up with the latest vulnerabilities and patches.
9. Stay Curious and Keep Learning
Technology evolves rapidly, and staying updated is essential.
- For Novices: Follow blogs, take online courses, and experiment with new languages or frameworks.
- For Veterans: Attend conferences, contribute to open-source projects, and stay curious.
Feature/Spec | Novice Developers | Veteran Developers |
---|---|---|
Code Readability | Use descriptive variable names | Write self-documenting code |
Error Handling | Basic try-catch blocks | Advanced logging and custom errors |
Testing | Manual and unit testing | Integration and automated testing |
Version Control | Commit, push, pull, and branching basics | Advanced Git workflows (rebasing, hooks) |
Documentation | Inline comments and basic guides | API documentation and structured guides |
Security | Basic practices (input sanitization) | Regular audits and proactive measures |
Collaboration | Participate in code reviews | Mentor, lead discussions, and code reviews |
Learning | Follow tutorials and experiment | Attend conferences and contribute to open-source |
Final Thoughts
The unspoken rules of coding serve as a guiding compass for developers at every stage of their journey. While novices should focus on building foundational habits, veterans must continually refine their craft and lead by example. By adhering to these principles, you not only elevate your own skills but also contribute to a culture of excellence in the software development community.
So, whether you’re writing your first “Hello, World!” or architecting a complex system, remember: coding is as much about discipline and communication as it is about creativity and problem-solving.
Read More= UndergrowthGameLine Hosted Event